Num Expression#
Functionality#
This node is currently fastest way to evaluate an expression. For best performance the node should get NumPy arrays. But vectorization is slow as it is for any other node in Sverchok, so make sure that you have all numbers in a single object if performance is important.
The node is based on NumExpr library. It supports next operators:
Bitwise operators (and, or, not, xor):
&, |, ~, ^
Comparison operators:
<, <=, ==, !=, >=, >
Unary arithmetic operators:
-
Binary arithmetic operators:
+, -, *, /, **, %, <<, >>
Supported functions:
where(bool, number1, number2): number
– number1 if the bool condition is true, number2 otherwise.
{sin,cos,tan}(float|complex): float|complex
– trigonometric sine, cosine or tangent.
{arcsin,arccos,arctan}(float|complex): float|complex
– trigonometric inverse sine, cosine or tangent.
arctan2(float1, float2): float
– trigonometric inverse tangent of float1/float2.
{sinh,cosh,tanh}(float|complex): float|complex
– hyperbolic sine, cosine or tangent.
{arcsinh,arccosh,arctanh}(float|complex): float|complex
– hyperbolic inverse sine, cosine or tangent.
{log,log10,log1p}(float|complex): float|complex
– natural, base-10 and log(1+x) logarithms.
{exp,expm1}(float|complex): float|complex
– exponential and exponential minus one.
sqrt(float|complex): float|complex
– square root.
abs(float|complex): float|complex
– absolute value.
conj(complex): complex
– conjugate value.
{real,imag}(complex): float
– real or imaginary part of complex.
complex(float, float): complex
– complex from real and imaginary parts.
contains(np.str, np.str): bool
– returns True for every string inop1
that containsop2
.
{floor}(float): float
– returns the greatest integer less than or equal to x.
{ceil}(float): float
– returns the least integer greater than or equal to x
Reduction functions:
sum(number, axis=None)
: Sum of array elements over a given axis. Negative axis are not supported.
prod(number, axis=None)
: Product of array elements over a given axis. Negative axis are not supported.
{min,max}(number, axis=None)
: Find minimum/maximum value over a given axis. Negative axis are not supported.
Note: because of internal limitations, reduction operations must appear the last in the stack. If not, it will be issued an error like:
>>> ne.evaluate('sum(1)*(-1)')
RuntimeError: invalid program: reduction operations must occur last
The node automatically generates sockets for each variable in an input formula.
The type of sockets can be either float/int or vector. The type of generated
socket is dependent on the name of the variable. To generate vector type the
variable should statr with upper V
letter. Any other variable names will
generate float/int type. The order of sockets is dependent on variable names.
They are sorted in alphabetical order. The output type is vector if there is
at least one input vector type. Variable names should be Pythonic.
Inputs#
Variable number of inputs depending on the input formula.
Outputs#
Result
Examples#
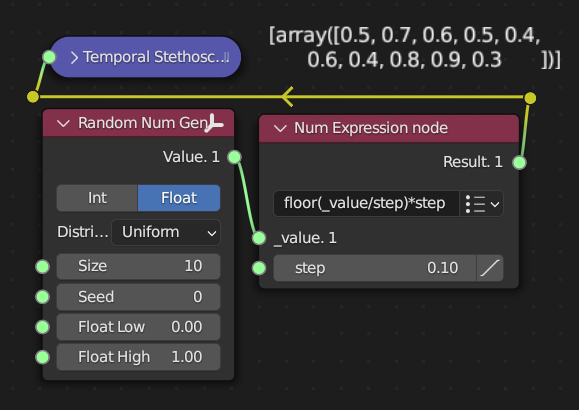
Round a value according to given step.#